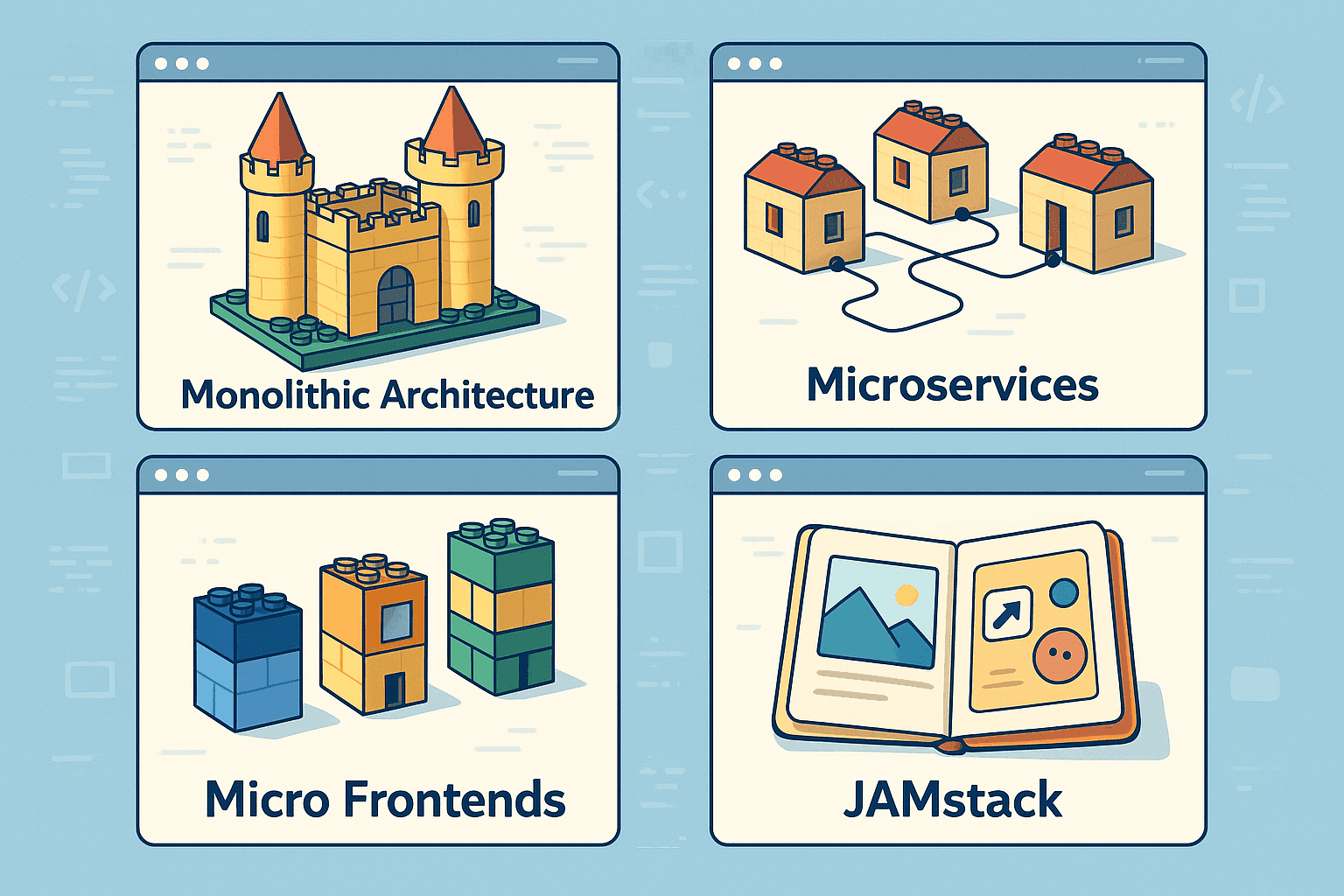
Choosing the right architecture for your web application's front-end is crucial. It's the blueprint that dictates how your user interface is built, how easily it can grow, and how efficiently your teams can work.
Think of it like building with LEGOs: you could randomly stick bricks together, but a plan ensures a sturdy, well-organized, and impressive final structure. The front-end is everything your users see and interact with – the buttons, text, and images. Just like different LEGO sets offer various building styles, front-end architectures provide distinct approaches to structuring this user-facing part of your application. Understanding these styles helps us create better digital experiences.
The One Big Castle: Monolithic Architecture
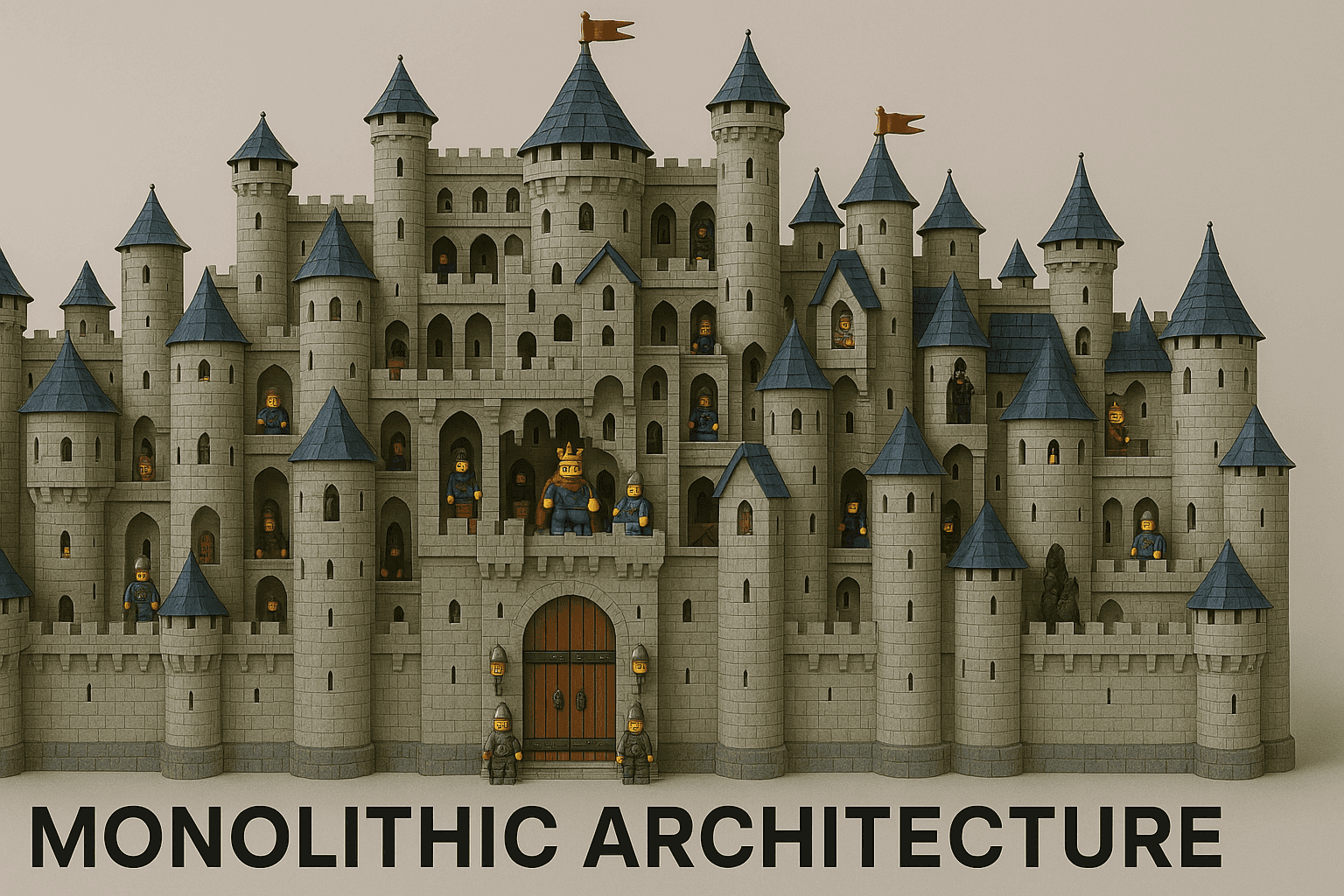
Imagine a single, large LEGO castle where every room, tower, and wall is interconnected. In a monolithic front-end architecture, all the UI components and logic are bundled together into one large codebase and deployment unit. Even if the backend is separate, the front-end itself is a single entity. Think of a traditional WordPress site where the theme handles most of the display logic in one place.
As Sam Newman, author of "Building Microservices," notes A monolithic architecture is a choice, and a valid one at that. It may not be the right choice in all circumÂstances...but it's a choice nonetheless.
Pros:
- Simpler Start: Generally easier to develop, test, and deploy initially, especially for small projects or teams. It's like having all your bricks in one box.
- Easy Code Sharing: Sharing code and managing communication between parts is straightforward within a single codebase.
- Initial Speed: Can be faster to get started for simple applications.
Cons:
- Scalability Challenges: Difficult to scale specific parts; you often have to scale the entire application.
- Technology Lock-in: Less flexibility to introduce different technologies for different parts.
- Deployment Risks: Small changes require redeploying the entire front-end, which can be slow and risky.
- Decreased Velocity Over Time: Development can slow down as the codebase grows and more developers contribute.
- Impact of Failures: A problem in one area can potentially bring down the entire front-end.
- Difficult to Modernize: Adopting new frameworks or major changes can be challenging.
Code Example (Conceptual TypeScript/React To-Do App):
This example shows a simple to-do list where all logic resides in a single component.
// src/App.tsx
import React, { useState } from 'react';
interface Todo {
id: number;
text: string;
}
const App: React.FC = () => {
const [todos, setTodos] = useState<Todo[]>([]); // State for the list of todos
const [newTodo, setNewTodo] = useState<string>(""); // State for the input field
const [nextId, setNextId] = useState<number>(1); // Simple ID generation
const handleInputChange = (event: React.ChangeEvent<HTMLInputElement>) => {
setNewTodo(event.target.value); // Update input field state
};
const handleAddTodo = () => {
if (newTodo.trim() !== "") {
const newTodoItem: Todo = { id: nextId, text: newTodo };
setTodos([...todos, newTodoItem]); // Add new todo to the list
setNewTodo(""); // Clear input field
setNextId(nextId + 1); // Increment ID for the next todo
}
};
return ( // Render the UI elements
<div>
<h1>My To-Do List</h1>
<input type="text" value={newTodo} onChange={handleInputChange} placeholder="Add a new task" />
<button onClick={handleAddTodo}>Add Todo</button>
<ul>
{todos.map(todo => (
<li key={todo.id}>{todo.text}</li>
))}
</ul>
</div>
);
};
export default App; // Export the main component
Use Cases: Small to medium-sized applications, projects with small development teams, MVPs (Minimum Viable Products), situations where rapid initial development is prioritized.
Many Small Rooms: Microservices (Applied to Front-End)
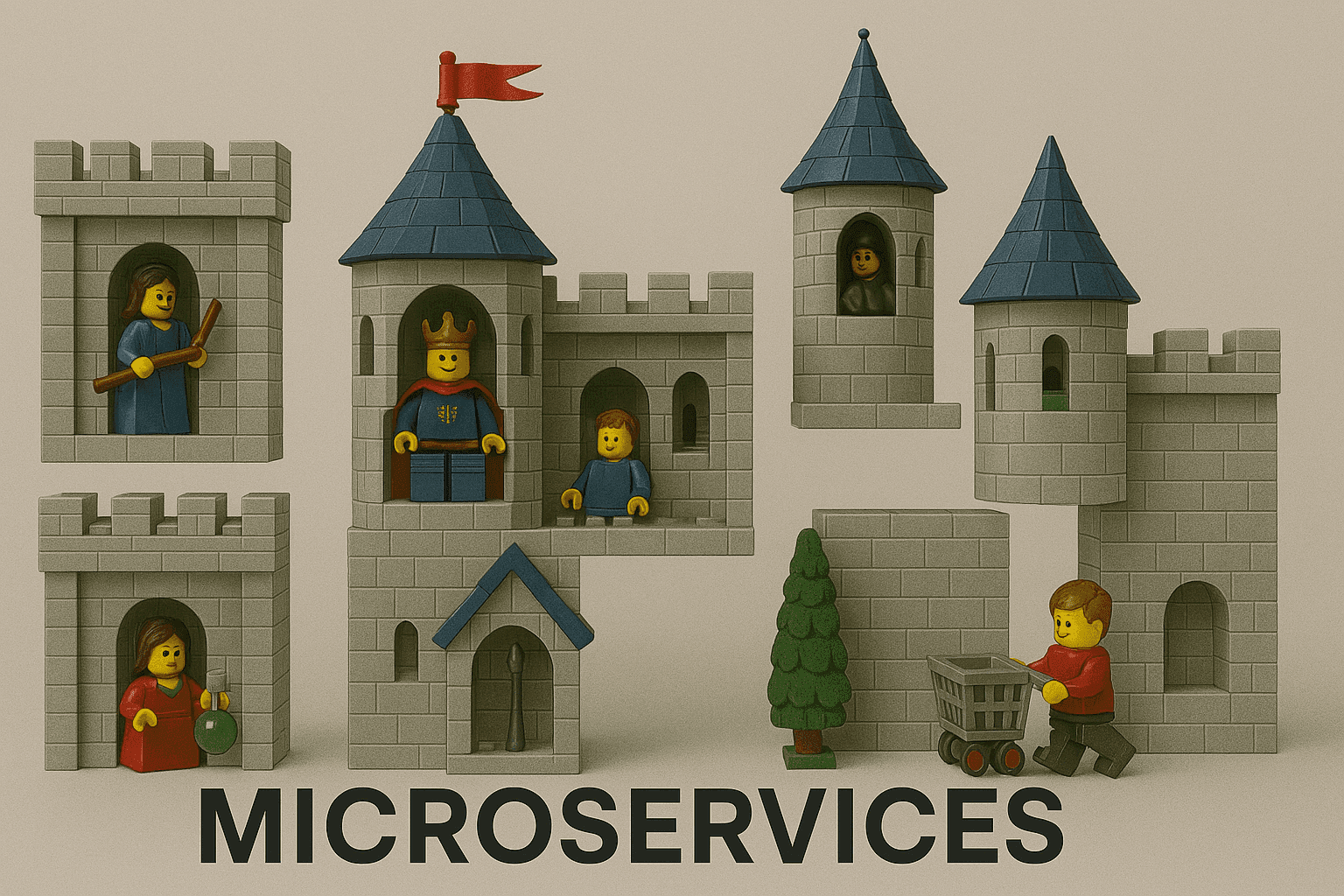
Imagine building that LEGO castle with separate, smaller rooms or modules that work independently but can communicate.
While microservices traditionally refer to backend architecture, the principles can influence the front-end.
This often involves the front-end interacting with multiple specialized backend microservices via APIs. Each backend service handles a distinct business capability (like user profiles, shopping carts), and the front-end integrates data from these sources.
As one article puts it, Microservices can be scaled independently based on demand... [leading] to better resource utilization... However, it also introduces new challenges that organizations must navigate.
Pros:
- Independent Backend Scaling: Backend services can be scaled independently based on need.
- Technology Flexibility (Backend): Different backend services can use different technologies.
- Improved Fault Isolation (Backend): Failure in one backend service is less likely to affect the entire system.
- Faster Backend Development Cycles: Backend teams can work independently on their services.
Cons (Primarily related to managing interactions):
- Complexity: Managing interactions with numerous backend services adds complexity to the front-end.
- Network Latency: Communication between the front-end and multiple backend services can introduce latency.
- Data Consistency: Ensuring data consistency across different sources can be challenging.
- Complex Testing: End-to-end testing involving multiple services is more complex.
- Infrastructure Overhead: Requires potentially more infrastructure for managing APIs and service communication.
Code Example (Conceptual API Interaction):
This shows a component fetching data from two different hypothetical backend services.
// src/UserDataDisplay.tsx
import React, { useEffect, useState } from 'react';
interface User { // Define User data structure
id: number;
name: string;
}
interface Product { // Define Product data structure
id: number;
name: string;
price: number;
}
// Simulated API call to a 'user' microservice
const fetchUserData = async (): Promise<User> => {
// In reality, this would be: const response = await fetch('/api/user/profile');
console.log("Fetching user data...");
return new Promise(resolve => setTimeout(() => resolve({ id: 1, name: 'Alice' }), 500));
};
// Simulated API call to a 'product' microservice
const fetchFavoriteProduct = async (): Promise<Product> => {
// In reality, this would be: const response = await fetch('/api/products/favorite/1');
console.log("Fetching product data...");
return new Promise(resolve => setTimeout(() => resolve({ id: 101, name: 'Super Widget', price: 29.99 }), 800));
};
const UserDataDisplay: React.FC = () => {
const [user, setUser] = useState<User | null>(null);
const [product, setProduct] = useState<Product | null>(null);
const [loading, setLoading] = useState<boolean>(true);
useEffect(() => {
const loadData = async () => {
try {
setLoading(true);
// Fetch data from different conceptual 'services'
const userData = await fetchUserData();
const favProduct = await fetchFavoriteProduct();
setUser(userData);
setProduct(favProduct);
} catch (error) {
console.error("Failed to fetch data:", error);
// Handle error appropriately
} finally {
setLoading(false);
}
};
loadData();
}, []); // Empty dependency array means this runs once on mount
if (loading) {
return <div>Loading data from services...</div>;
}
return (
<div>
<h2>User Profile</h2>
{user ? <p>Name: {user.name}</p> : <p>User data not found.</p>}
<h2>Favorite Product</h2>
{product ? <p>Name: {product.name} - Price: ${product.price}</p> : <p>Product data not found.</p>}
</div>
);
};
export default UserDataDisplay;
Use Cases: Applications with complex backend logic divided into microservices, scenarios requiring high backend scalability and resilience.
Tiny Independent Blocks: Micro Frontends
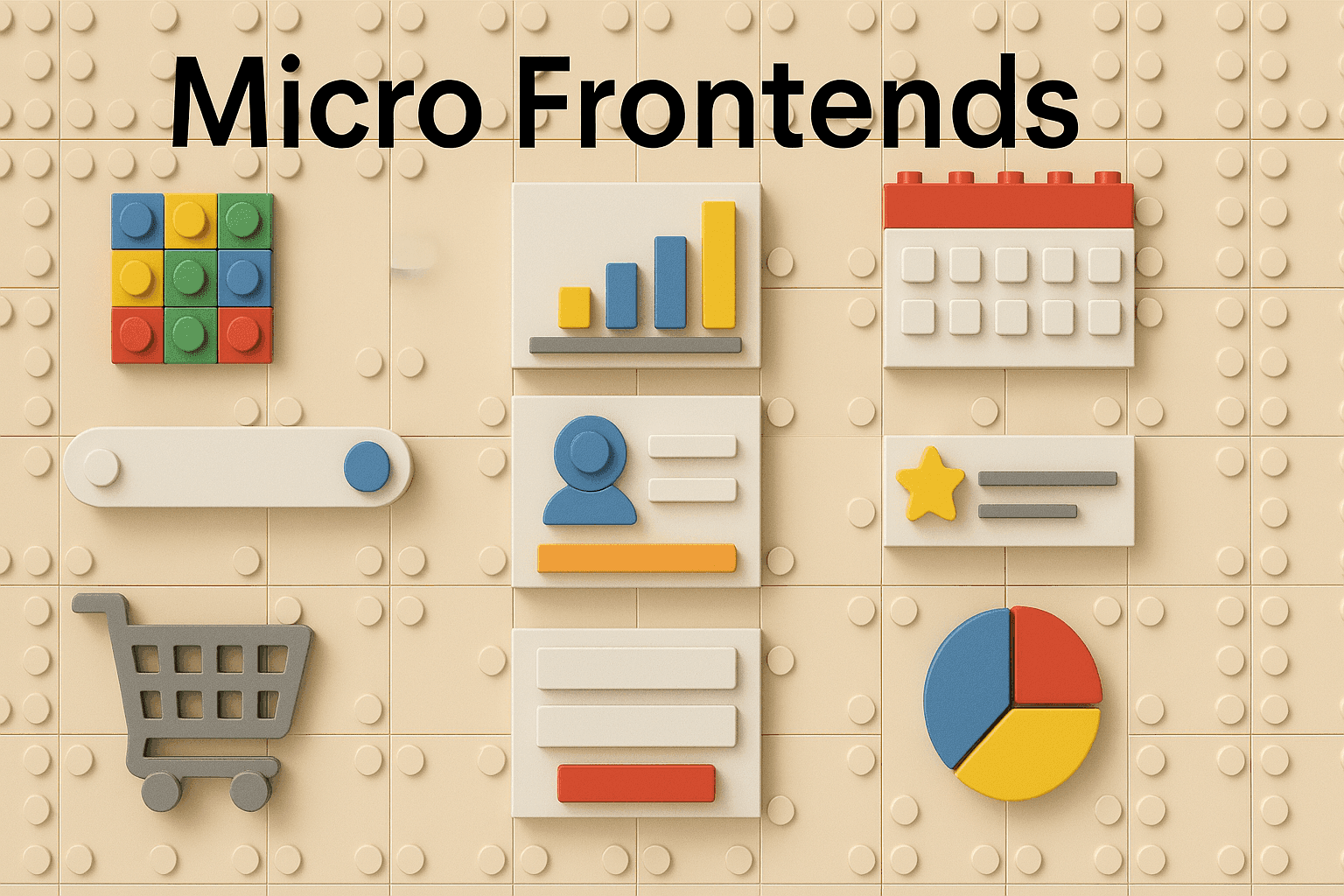
Let's take the idea of separation further. Imagine tiny, specific LEGO blocks, each representing a distinct feature or UI section, built and managed by different teams. These blocks can be developed, tested, and deployed independently without impacting others. This is the core idea of Micro Frontends (MFE).
According to micro-frontends.org, The idea behind Micro Frontends is to think about a website or web app as a composition of features which are owned by independent teams... A team is cross functional and develops its features end-to-end, from database to user interface.
Different teams might even use different frameworks (React, Vue, Angular) for their specific micro frontend. A container application (sometimes called a shell) orchestrates how these independent pieces fit together to create a cohesive user experience.
Pros:
- Team Autonomy: Teams can develop, test, and deploy their features independently.
- Technology Flexibility: Different teams can choose the best stack for their specific feature.
- Scalable Development: Easier to scale development efforts across multiple teams working in parallel.
- Faster, Focused Releases: Features can be released more quickly and independently.
- Smaller Codebases: Individual micro frontends have smaller, more manageable codebases.
- Gradual Modernization: Allows for incremental upgrades or rewrites of legacy systems.
Cons:
- Integration Complexity: Orchestrating the different micro frontends and ensuring smooth communication/navigation between them can be complex. Clear contracts (APIs) are needed.
- Potential Performance Overhead: Loading multiple frameworks or duplicated code can increase payload size and impact performance if not managed carefully.
- Operational Complexity: Requires more sophisticated CI/CD pipelines and infrastructure to manage independent deployments.
- Maintaining Consistency: Ensuring a consistent look, feel, and user experience across different parts built by different teams requires strong design systems and coordination.
- Shared Dependencies: Managing shared libraries or state across micro frontends needs careful consideration.
Code Example: A true micro frontend code example is more complex, often involving techniques like module federation (Webpack 5+), iframes, or web components combined with a container application. These are beyond simple snippets. You can explore examples and patterns on resources like micro-frontends.org or search for specific implementations like "Webpack Module Federation example."
Use Cases: Large, complex web applications developed by multiple autonomous teams, organizations wanting to migrate legacy front-ends incrementally, scenarios where technology diversity across features is desired or necessary.
Super Fast Static Pictures with Dynamic Stickers: JAMstack
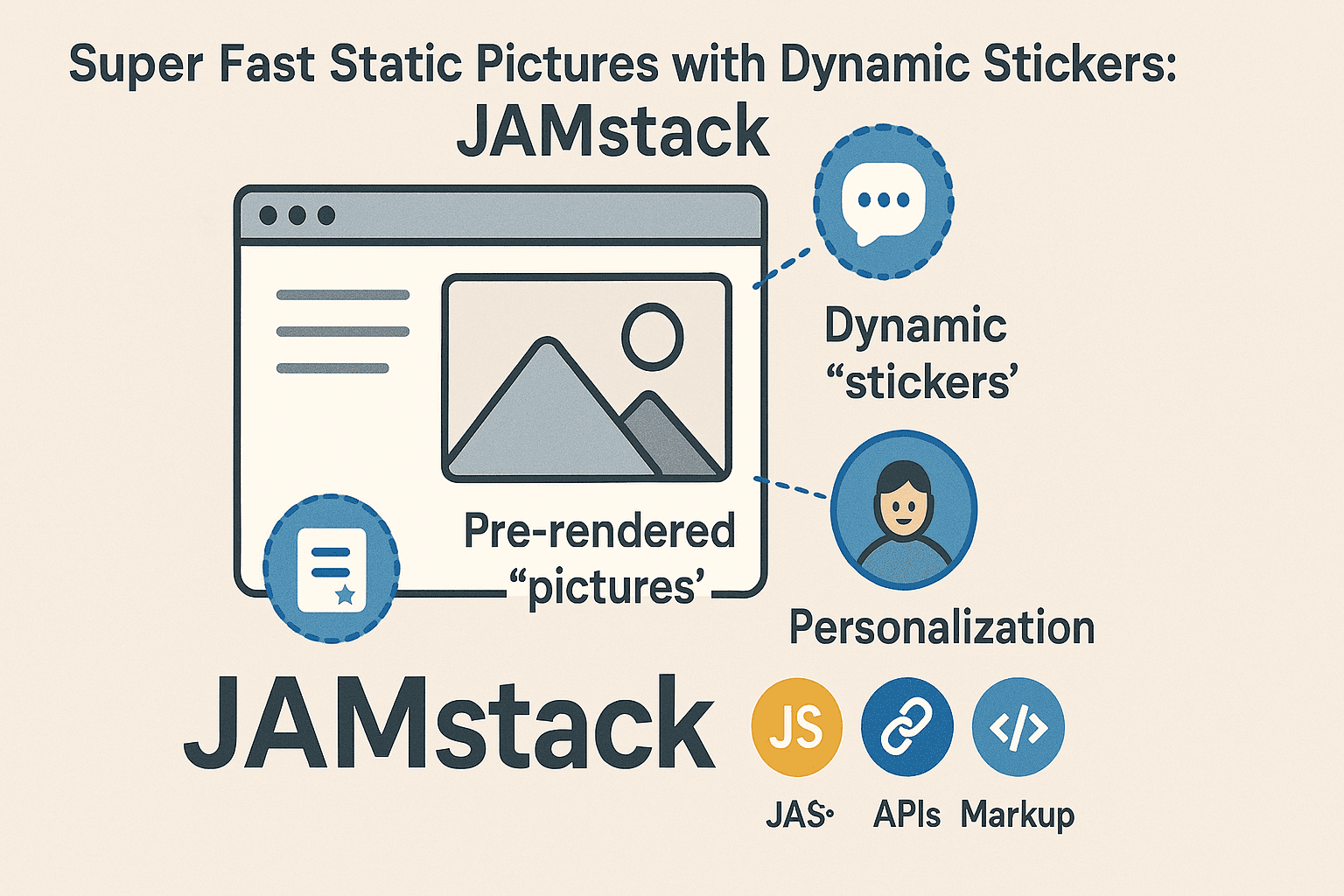
Think of a website built mostly from pre-rendered "pictures" (static HTML files) that load incredibly fast, like flipping through a photo album. This pre-built Markup forms the core structure. Dynamic elements or interactions (like user comments, forms, personalization) are added as "stickers" using client-side JavaScript that talks to backend services via APIs.
This is JAMstack: JavaScript, APIs, and Markup. The focus is on pre-rendering as much as possible during a build step and serving static assets globally via Content Delivery Networks (CDNs).
One source highlights that The Jamstack architecture has changed the way web development is done by putting speed, security, and scalability at the top of the list.
Pros:
- Performance: Extremely fast load times due to pre-built files served from CDNs.
- Security: Reduced attack surface as there's often no direct database or server-side code execution exposed for static parts.
- Scalability: Static files scale effortlessly on CDNs to handle high traffic.
- Developer Experience: Often involves modern tooling, Git-based workflows, and automated builds.
- Lower Cost: Hosting static files on CDNs can be significantly cheaper than running traditional servers.
- SEO: Search engines can easily crawl and index pre-rendered static content.
Cons:
- Dynamic Content Complexity: Handling highly dynamic or real-time features requires client-side JavaScript and reliance on APIs or serverless functions, which can add complexity.
- Build Times: For very large sites, build times (pre-rendering) can become long.
- Learning Curve: May require familiarity with specific static site generators (e.g., Next.js, Gatsby, Hugo), build tools, and serverless concepts.
- Dependency on Services: Relies on third-party APIs and services for dynamic functionality.
- Not Ideal for Real-time: Less suitable for applications requiring constant, real-time data updates across many users without complex client-side logic.
Code Example:
A JAMstack example typically involves a Static Site Generator (SSG) like Next.js or Gatsby. The code would show configuration for pre-rendering pages and potentially useEffect
hooks in React components to fetch dynamic data from APIs after the initial static load. You can find starter templates and examples on the official websites for these frameworks (Next.js, Gatsby).
Use Cases: Blogs, documentation sites, marketing websites, portfolios, e-commerce storefronts (where the catalogue is static but cart/checkout is dynamic), landing pages – essentially many sites where content is not constantly changing in real-time and performance/security are key.
Comparative Overview
Feature | Monolithic | Microservices (Front-End Interaction) | Micro Frontends | JAMstack |
---|---|---|---|---|
Initial Complexity | Low | Moderate (API integration) | High (Orchestration, Integration) | Low to Moderate (Tooling) |
Scalability | Lower (Scale whole app) | High (Backend services) | High (Independent features) | Very High (CDN) |
Team Independence | Low | Moderate (Backend teams) | High (Feature teams) | Moderate (Depends on API providers) |
Tech Flexibility | Low | High (Backend) | Very High (Per MFE) | Moderate (Client-side JS, APIs) |
Deployment | Single Unit, Slower/Riskier | Independent (Backend services) | Independent (Per MFE), Complex CI/CD | Simple (Static files), Fast Build/Deploy |
When to Choose Which Architecture?
- Monolithic: Best for smaller projects, MVPs, small/single teams, or when initial speed is paramount and complexity is low.
- Microservices (Front-End Interaction): Suitable when your backend is already built as microservices and the front-end needs to integrate with these diverse capabilities.
- Micro Frontends: Ideal for large, complex applications managed by multiple independent teams needing autonomy and technology flexibility. Excellent for gradually modernizing large monoliths.
- JAMstack: Perfect for sites prioritizing performance, security, and scalability, like blogs, marketing sites, docs, and e-commerce storefronts. Less ideal for highly dynamic, real-time applications.
Why is Choosing the Right Architecture Interesting?
Selecting the appropriate front-end architecture significantly impacts your project's success. It influences:
- Maintainability: How easy is it to fix bugs and update features?
- Scalability: Can the application handle growth in users and complexity?
- Team Productivity: Can teams work independently and efficiently?
- Performance & User Experience: How fast and responsive is the application for the end-user?
- Technology Adoption: How easily can you adopt new tools and frameworks?
Making an informed decision early on prevents significant refactoring pain later and empowers your team to build better products faster.
SEO Keywords
- Front-End Architecture
- Micro Frontends
- JAMstack
- Monolithic Architecture
- Web Development Patterns
Sources and Further Reading
- Micro Frontends Info: micro-frontends.org
- JAMstack Info: Jamstack.org
- Next.js (React Framework/SSG): Official Next.js Docs
- Gatsby (React Framework/SSG): Official Gatsby Docs
- Goodreads (Quotes from "Monolith to Microservices"): Goodreads Quotes Page
- Elitex Guide on Micro Frontends: Micro Frontend Architecture: A Full 2025 Guide - ELITEX
- HackerNoon on Micro Frontends: Understanding Micro Frontends | HackerNoon
- BM Coder on Jamstack: Jamstack development - BM coder
- eSparkBiz on Jamstack Pros/Cons: Pros and Cons of JAMSTACK For The Year 2025 - eSparkBiz
- Medium Article on Microservices: Microservices Architecture: Benefits and Challenges | by Milad Fahmy - Medium
- Startechup on Microservices Challenges: 8 Microservices Challenges and How to Overcome Them - Startechup